Read Email and Parse Email in C# - TutorialEAGetMail is an email component which supports all operations of POP3/IMAP4/MIME/Exchange Web Service/WebDAV/S/MIME/SSL protocol. This tutorial introduces how to read email in C# using POP3/IMAP4/EWS/WebDAV protocol and parse email body, attachment. It also demonstrates how to decrypt email and verify email digital signature (S/MIME) in C#.
read original tutorialTutorial Index:Read email in a simple C# project using POP3
Read email from IMAP4 server
Read email over SSL connection
Read email from Gmail account
Read email from Yahoo account
Read email from Hotmail/MSN Live account
Read email with event handler
Using UIDL function to mark the email has been downloaded
Read email with background service
Parse email
Verify digital signature and decrypt email - S/MIME
Parse winmail.dat (TNEF)
Convert email to HTML page and display it using Web browser
Parse Non-delivery report (NDS)
Sample projects
InstallationBefore you can use the following sample codes, you should download the
EAGetMail Installer and install it on your machine at first.
Read email in a simple C# projectTo better demonstrate how to read and parsing email, let's create a C# console project named "receiveemail" at first, and then add the reference of EAGetMail in your project.
Add Reference of EAGetMail to Visual Stuido C#.NET ProjectTo use EAGetMail in your project, the first step is "Add reference of EAGetMail to your project". Please create/open your project with Visual Studio.NET, then choose menu->"Project"->"Add Reference"->".NET"->"Browse...", and choose the EAGetMail{version}.dll from your disk, click "Open"->"OK", the reference of EAGetMail will be added to your project, and you can start to use EAGetMail POP3 & IMAP Component in your project.
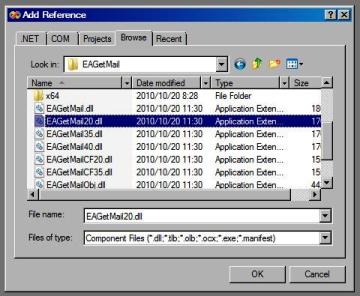
Because EAGetMail has separate builds for .Net Framework, please refer to the following table and choose the correct dll.
Separate builds of run-time assembly for .Net Framework 1.1, 2.0, 3.5, 4.0 and .Net Compact Framework 2.0, 3.5.
EAGetMail.dll Built with .NET Framework 1.1
It requires .NET Framework 1.1, 2.0, 3.5 or later version.
EAGetMail20.dll Built with .NET Framework 2.0
It requires .NET Framework 2.0, 3.5 or later version.
EAGetMail35.dll Built with .NET Framework 3.5
It requires .NET Framework 3.5 or later version.
EAGetMail40.dll Built with .NET Framework 4.0
It requires .NET Framework 4.0 or later version.
EAGetMailCF20.dll Built with .NET Compact Framework 2.0
It requires .NET Compact Framework 2.0, 3.5 or later version.
EAGetMailCF35.dll Built with .NET Compact Framework 3.5
It requires .NET Compact Framework 3.5 or later version.
[C# - Read email from POP3 server]Now add the following codes to the project. The following code demonstrates how to read email from a POP3 mail account. This sample downloads emails from POP3 server and deletes the email after the email is retrieved.
using System;
using System.Collections.Generic;
using System.Text;
using System.IO;
using EAGetMail; //add EAGetMail namespace
namespace receiveemail
{
class Program
{
static void Main(string[] args)
{
// Create a folder named "inbox" under current directory
// to save the email retrieved.
string curpath = Directory.GetCurrentDirectory();
string mailbox = String.Format("{0}\\inbox", curpath);
// If the folder is not existed, create it.
if (!Directory.Exists(mailbox))
{
Directory.CreateDirectory(mailbox);
}
MailServer oServer = new MailServer("pop3.emailarchitect.net",
"test@emailarchitect.net", "testpassword", ServerProtocol.Pop3 );
MailClient oClient = new MailClient("TryIt");
// If your POP3 server requires SSL connection,
// Please add the following codes:
// oServer.SSLConnection = true;
// oServer.Port = 995;
try
{
oClient.Connect(oServer);
MailInfo[] infos = oClient.GetMailInfos();
for (int i = 0; i < infos.Length; i++)
{
MailInfo info = infos[i];
Console.WriteLine("Index: {0}; Size: {1}; UIDL: {2}",
info.Index, info.Size, info.UIDL);
// Read email from POP3 server
Mail oMail = oClient.GetMail(info);
Console.WriteLine("From: {0}", oMail.From.ToString());
Console.WriteLine("Subject: {0}\r\n", oMail.Subject);
// Generate an email file name based on date time.
System.DateTime d = System.DateTime.Now;
System.Globalization.CultureInfo cur = new
System.Globalization.CultureInfo("en-US");
string sdate = d.ToString("yyyyMMddHHmmss", cur);
string fileName = String.Format("{0}\\{1}{2}{3}.eml",
mailbox, sdate, d.Millisecond.ToString("d3"), i);
// Save email to local disk
oMail.SaveAs(fileName, true);
// Mark email as deleted from POP3 server.
oClient.Delete(info);
}
// Quit and pure emails marked as deleted from POP3 server.
oClient.Quit();
}
catch (Exception ep)
{
Console.WriteLine(ep.Message);
}
}
}
}
If you set everything right, you can get emails in the mail folder. If the codes threw exception, then please have a look at the following section:
Where can I get my POP3 server address, user and password?Because each email account provider has different server address, so you should query your POP3 server address from your email account provider. User name is your email address or your email address without domain part. It depends on your email provider setting.
When you execute above example code, if you get error about "Networking connection" or "No such host", it is likely that your POP3 server address is not correct. If you get an error like "Invalid user or password", it is likely that you did not set the correct user or password.
Finally, if you have already set your account in your email client such as Outlook or Window Mail, you can query your POP3 server address, user in your email client. For example, you can choose menu -> "Tools" - > - "Accounts" - > "Your email account" - > "Properties" - > "Servers" in Outlook express or Windows Mail to get your POP3 server, user. Using EAGetMail to receive email does not require you have email client installed on your machine or MAPI, however you can query your exist email accounts in your email client.
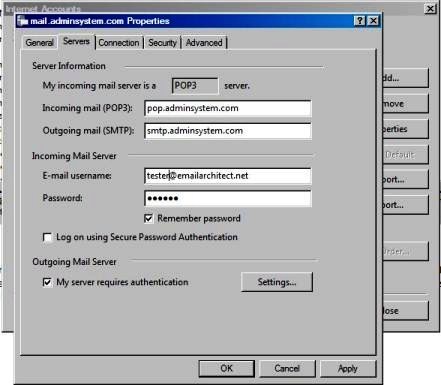
Edited by user Wednesday, July 24, 2013 1:54:27 AM(UTC)
| Reason: Not specified